Understanding Idempotence in APIs for Web Development
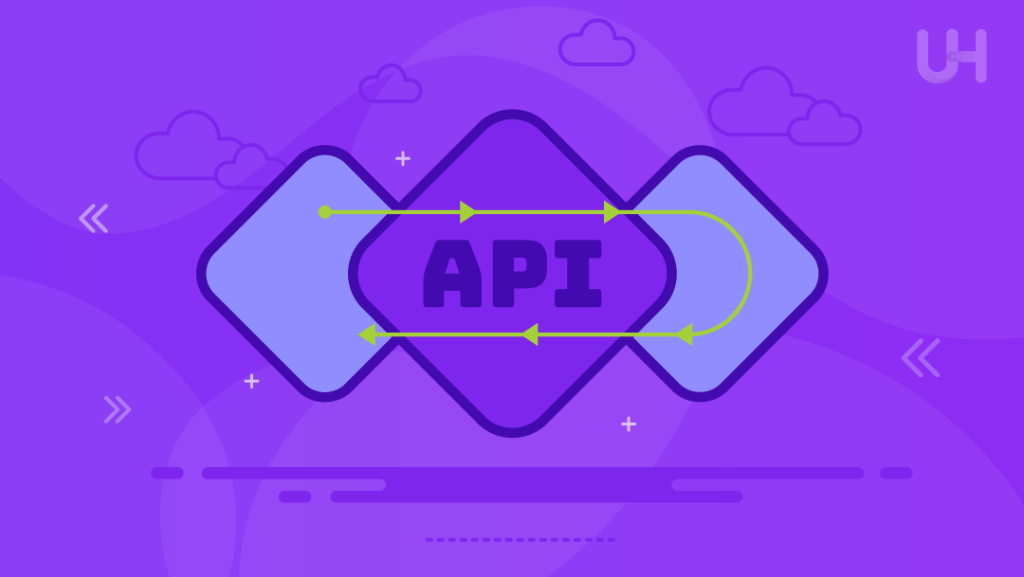
When building modern web applications, especially those that handle sensitive operations like financial transactions, bookings, or data processing, one of the core principles developers often implement is idempotence. Idempotence ensures that a system behaves predictably even if an operation is repeated multiple times. For APIs, idempotence provides consistency, safety, and reliability in request handling. This article dives into the concept of idempotence in APIs, why it’s essential in web development, and how to implement it effectively.
What is Idempotence?
In the context of web development and APIs, idempotence means that a particular operation can be performed multiple times without changing the result beyond the initial application. This concept, often used in mathematical and functional programming contexts, ensures that a repeated operation produces the same effect as a single invocation.
For example, consider an API endpoint that is designed to create a resource, such as a payment. If you call this endpoint more than once with the same request data, idempotence guarantees that only one payment will be processed. All subsequent calls will have no additional effect.
Why Idempotence is Important for APIs
Idempotence is essential for APIs, particularly for these scenarios:
- Preventing Duplicate Operations: It’s common for network failures, timeouts, or user impatience to result in the same request being sent multiple times. Idempotent APIs prevent these duplicates from causing unintended consequences, such as processing multiple payments.
- Improving Fault Tolerance: In distributed systems, requests may be retried if they fail due to network issues or backend unavailability. An idempotent API ensures that retries do not cause multiple state changes.
- Maintaining Data Integrity: Ensuring data consistency is critical, especially when dealing with financial data or transactions. Idempotent operations help avoid data corruption, overcharges, or duplicate records.
HTTP Methods and Idempotency
Some HTTP methods are inherently idempotent, while others are not. Here’s a quick breakdown of how common HTTP methods relate to idempotency:
- GET: Naturally idempotent. Fetching data from an endpoint multiple times does not change any server state.
- PUT: Should be idempotent by design. It replaces or updates a resource entirely. Multiple PUT requests with the same payload should produce the same end state.
- DELETE: Generally idempotent. Deleting a resource multiple times should yield the same result—no further action after the initial deletion.
- POST: Not inherently idempotent. POST requests usually create new resources or trigger actions, so repeated requests can result in duplicate resources.
Making POST Idempotent
Since POST is not inherently idempotent but often required for actions that should be idempotent (e.g., creating a unique transaction), developers can implement idempotency for POST in two ways:
- Idempotency Keys: The client includes a unique key (idempotency key) with each request. The server then uses this key to track the request and ensure that any repeated request with the same key does not result in a duplicated action.
- Idempotent Resource Creation Logic: Instead of treating POST requests as pure create operations, you might use resource attributes (e.g., transaction ID) to verify if the request is indeed a duplicate.
How to Implement Idempotency in API Design
Implementing idempotency in your API is about managing state changes carefully. Here’s a step-by-step approach:
1. Use Idempotency Keys
- When designing POST requests for critical actions (e.g., payments or bookings), allow clients to send a unique idempotency key.
- Store the idempotency key along with the request metadata in your backend.
- If the same idempotency key is received again, check the stored metadata and return the previous result, avoiding the re-execution of the operation.
2. Store Request Metadata Temporarily
- Save the incoming request’s metadata temporarily, including details like status, request body, and response.
- Store these records for a limited time (based on business needs) to handle retries without occupying unnecessary storage.
3. Ensure Resource Identification
- Implement unique identifiers (UUIDs) for resources that need strict idempotency.
- Use these identifiers to validate if a resource has already been created, allowing the server to respond with the existing data without duplicating the resource.
4. Use Database Locks for State Changes (When Necessary)
- In scenarios where multiple concurrent requests may modify shared resources, database locks or transactions can help avoid unintended modifications.
- Use transaction management and ACID-compliant databases to ensure that only one operation is processed at a time for the affected resource.
Benefits of Using Idempotency in APIs
- Consistency and Reliability: Idempotency ensures that clients receive predictable responses even if they accidentally send duplicate requests.
- Data Integrity: For financial transactions or bookings, idempotency prevents double processing, safeguarding against overcharges and double-booking.
- Enhanced User Experience: Users can refresh or retry operations without concerns about creating unwanted duplicates.
Challenges and Best Practices
While implementing idempotency, developers should be mindful of a few challenges:
- Handling Expired Idempotency Keys: Decide how long to retain idempotency records. If a request is retried after a significant time, it may be allowed to reprocess.
- Performance Considerations: Storing idempotency keys and request metadata can be resource-intensive, especially in high-frequency systems. Consider database performance optimization and cleanup policies.
- Transaction Management: When applying idempotency to complex systems, use database transactions carefully to ensure atomic operations and avoid partial updates.
Idempotency in APIs is a powerful principle that enhances reliability and user experience in web applications, particularly when handling critical operations. By ensuring that repeated requests do not lead to duplicate actions, developers can build safer, more consistent APIs. Applying idempotency through careful design, such as using unique keys, consistent state management, and resource locks, provides the stability required in a robust web application.
Embracing idempotence as a standard practice for your API design allows for greater scalability, fault tolerance, and trustworthiness, especially in high-stakes web applications.
References:
https://www.freecodecamp.org/news/idempotency-in-http-methods/
https://blog.hubspot.com/website/idempotent-api
https://dev.to/karishmashukla/building-resilient-systems-with-idempotent-apis-5e5p